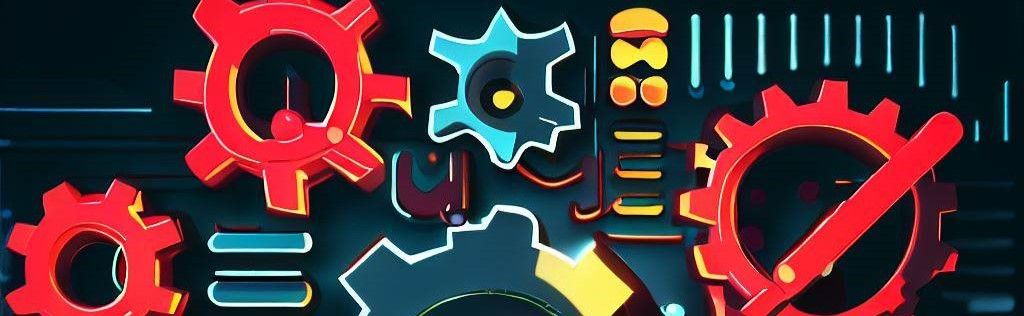
Unit Testing in C# with FakeItEasy and Fluent Assertions
Introduction: Why Unit Testing?
Untested code is broken code.
๐งจ Period. ๐งจ
If you're not unit testing your applications, you're leaving room for bugs, making future changes harder, and possibly, compromising the quality of your software.
In this article, we'll delve into the art of unit testing in C#, focusing on the libraries FakeItEasy for mocking and Fluent Assertions for assertive, readable checks.
My Personal Experience with Unit Testing
When I first encountered unit testing, I was skeptical. It seemed like writing tests would almost double the amount of code I'd have to maintain. Why spend that extra time on something that wasn't a core feature of the application? ๐คทโโ๏ธ
Fast forward six months, a client requested some changes to the application. Thanks to the unit tests, I could make those changes swiftly and verify that everything still worked as intended. No new bugs were introduced, and what could have taken hours of manual testing and troubleshooting was done in a fraction of the time.
The "extra" time spent writing tests initially had saved me hours of work later on. ๐
Benefits of Unit Testing
Before we jump into the technical aspects, let's understand why unit testing is crucial:
- Code Quality: Well-tested code usually equates to high-quality code.
- Maintainability: Unit tests act as a safety net, making future changes less risky.
- Documentation: Unit tests can serve as examples, thereby explaining how the code works.
- Confidence: The more you test, the more confident you are in your code's robustness.
Getting Started with Unit Testing in Visual Studio
To kick things off, you'll need to set up a test project in Visual Studio. Here's how:
- Create a new Project and choose "xUnit Test Project (.NET Core)" or "MSTest Project (.NET Core)".
- Add a reference to the project you're testing.
- Install the necessary packages, like FakeItEasy and Fluent Assertions.
Writing Your First Unit Test
Let's start by writing a basic test for a Calculator class, which has a method to add two integers.
// Calculator class
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
}
And here is how you would write a unit test for this method:
using FluentAssertions;
using Xunit;
public class CalculatorTests
{
[Fact]
public void Add_ReturnsCorrectSum()
{
// Arrange
var calculator = new Calculator();
// Act
int result = calculator.Add(2, 3);
// Assert
result.Should().Be(5);
}
}
Using FakeItEasy to Mock Dependencies
FakeItEasy helps in creating "fakes" or mocks for your dependencies. This makes testing in isolation easier. Let's say we have an `IOrderService` interface that we want to mock:
// Using FakeItEasy to create a fake object
var fakeOrderService = A.Fake<IOrderService>();
// Set up a fake behavior
A.CallTo(() => fakeOrderService.GetOrderById(1)).Returns(new Order { Id = 1, TotalPrice = 100 });
// Use the fake in test
fakeOrderService.GetOrderById(1).TotalPrice.Should().Be(100);
Conclusion
Unit testing is a must for any serious development effort. It offers an array of benefits from improving code quality to making your software maintainable.
FakeItEasy and Fluent Assertions make the process even more straightforward and enjoyable. Happy Testing!
- Hits: 650